A cheat sheet for Git CLI
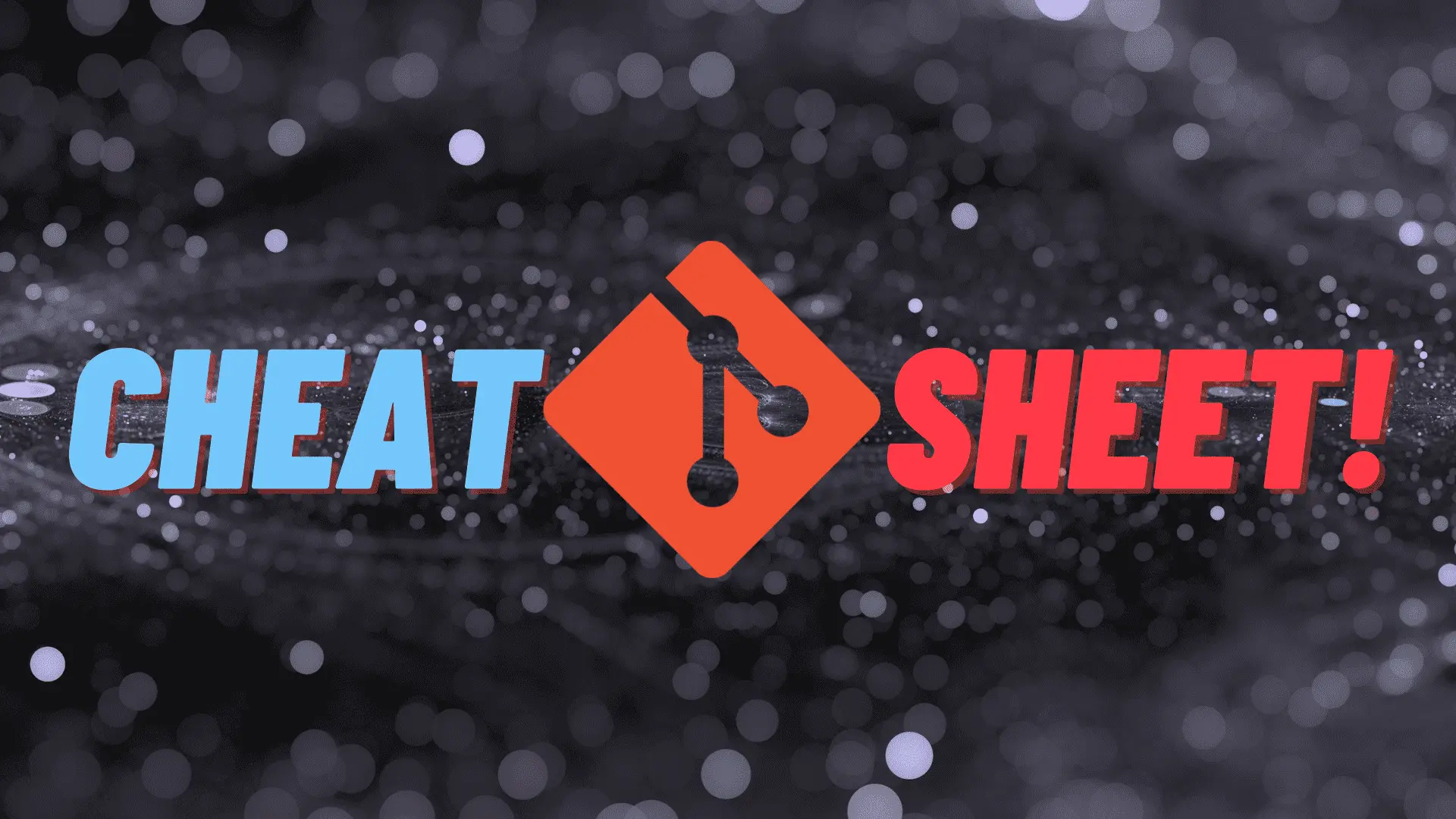
This is a cheat sheet for Git.
Setup
Configuring user information used across all local repositories
|
|
Setup & Init
Configuring user information, initializing and cloning repositories
Initialize an existing directory as a Git repository
|
|
Retrieve an entire repository from a hosted location via URL
|
|
Stage & Snapshot
Working with snapshots and the Git staging area
Show modified files in working directory, staged for your next commit
|
|
Add a file as it looks now to your next commit (stage)
|
|
Add all files as it looks now to your next commit (stage)
|
|
Unstage a file while retaining the changes in working directory
|
|
Diff of what is changed but not staged
|
|
Diff of what is staged but not yet commited
|
|
Commit your staged content as a new commit snapshot
|
|
Branch & Merge
Isolating work in branches, changing context, and integrating changes
List your branches. a * will appear next to the currently active branch
|
|
Create a new branch at the current commit
|
|
Switch to another branch and check it out into your working directory
|
|
Merge the specified branch’s history into the current one
|
|
Show all commits in the current branch’s history
|
|
Inspect & Compare
Examining logs, diffs and object information
Show the commit history for the currently active branch
|
|
Show the commits on branch A that are not on branch B
|
|
Show the commits that changed file, even across renames
|
|
Show the diff of what is in branchA that is not in branchB
|
|
Show any object in Git in human-readable format
|
|
Share & Update
Retrieving updates from another repository and updating local repos
Add a git URL as an alias
|
|
Fetch down all the branches from that Git remote
|
|
Merge a remote branch into your current branch to bring it up to date
|
|
Transmit local branch commits to the remote repository branch
|
|
Fetch and merge any commits from the tracking remote branch
|
|
Tracking path changes
Versioning file removes and path changes
Delete the file from project and stage the removal for commit
|
|
Change an existing file path and stage the move
|
|
Show all commit logs with indication of any paths that moved
|
|
Rewrite history
Rewriting branches, updating commits and clearing history
Apply any commits of current branch ahead of specified one
|
|
Clear staging area, rewrite working tree from specified commit
|
|
Discard changes in working directory if not staged yet
|
|
Temporary commits
Temporarily store modified, tracked files in order to change branches
Save modified and staged changes
|
|
List stack-order of stashed file changes
|
|
Write working from top of stash stack
|
|
Discard the changes from top of stash stack
|
|
Ignoring Patterns
Preventing unintentional staging or commiting of files
Save a file with desired paterns as .gitignore with either direct string matches or wildcard globs.
|
|
System wide ignore patern for all local repositories
|
|
**Source : ** https://education.github.com/git-cheat-sheet-education.pdf