A cheat sheet for Terraform CLI
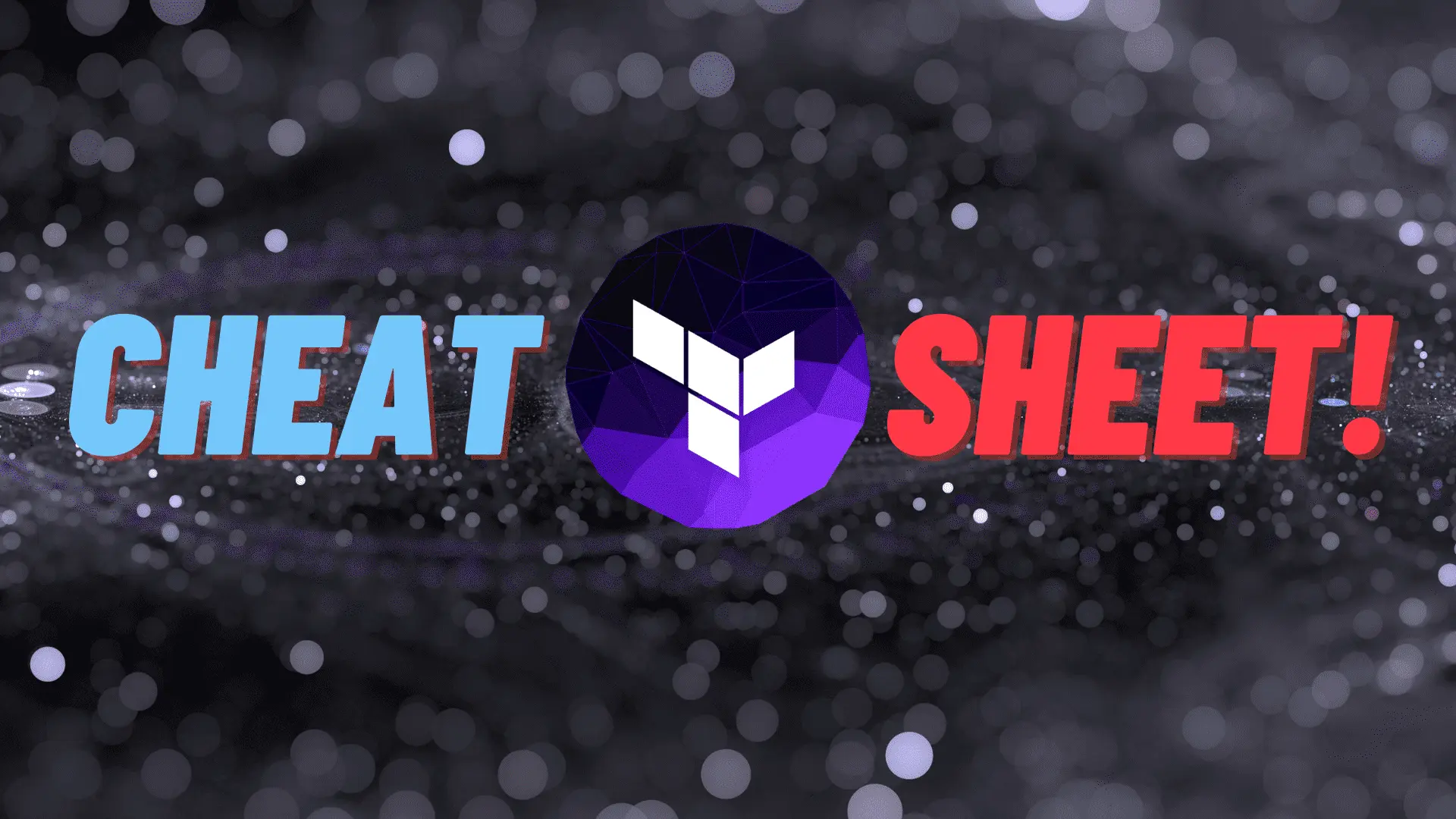
This is a cheat sheet for Terraform.
Terraform CLI Bash
Setup tab auto-completion, requires logging back in
|
|
Format and Validate Terraform Code
Format code
|
|
Validate code for synthax errors
|
|
Validate code without checking backend validation
|
|
Terraform Init
Initialize Terraform in current directory, pull down providers
|
|
Initialize Terraform in current directory, do not pull down providers
|
|
Initialize Terraform in current directory, do not verify Hashicorp signature
|
|
Plan, Apply and Utilities
Apply changes without being prompted to enter “yes”
|
|
Destroy infrastructure without being prompted to enter “yes”
|
|
Output the plan to a file : plan.out
|
|
Plan a destroy
|
|
Apply on a target resource
|
|
Variables into command
|
|
Lock : the state file so it can’t be modified by any other Terraform apply or modification action (possible only where backend allows locking)
|
|
Refresh : Do not reconcile state file with real-world resources(helpful with large complex deployments for saving deployment time)
|
|
Refresh : Reconcile the state in Terraform state file with real-world resources
|
|
Parallelism : Number of simultaneous resource operations
|
|
Providers : get informations about the current providers
|
|
Terraform Workspaces
Create a new workspace
|
|
Switch to another workspace
|
|
List all workspaces
|
|
Terraform State
Show details stored in Terraform state for the resource
|
|
Pull terraform state to a file
|
|
Move a resource tracked via state to different module
|
|
Replace an existing provider with another
|
|
List out all the resources tracked via the current state file
|
|
Remove a resource from the state, it doesn’t delete the resource
|
|
Terraform Import
Import EC2 instance
|
|
Import EC2 instance with a count
|
|
Terraform Outputs
List all outputs as stated in code
|
|
List out a specific declared output
|
|
List all outputs in JSON format
|
|
Terraform Taint
Taint resource to be recreated on next apply
|
|
Remove taint from a taint resource
|
|
Terraform Console
echo an expression into terraform console and see its expected result as output
|
|
Terraform console also has an interactive CLI just enter “terraform console”
|
|
Display the Public IP against the “my_ec2” Terraform resource as seen in the Terraform state file
|
|
Terraform Graph
Produce a PNG diagrams showing relationship and dependencies
|
|
Terraform Cloud
Obtain and save API token for Terraform cloud
|
|
Log out of Terraform Cloud, defaults to hostname app.terraform.io
|
|
Terraform Miscelleneous
Show version
|
|
Download and update modules in the “root” module.
|
|